Popovers are finally(!) possible on iPhone on iOS 8. The catch is that by default, they get presented modally over the full screen — not exactly what I’d call a ‘popover’.
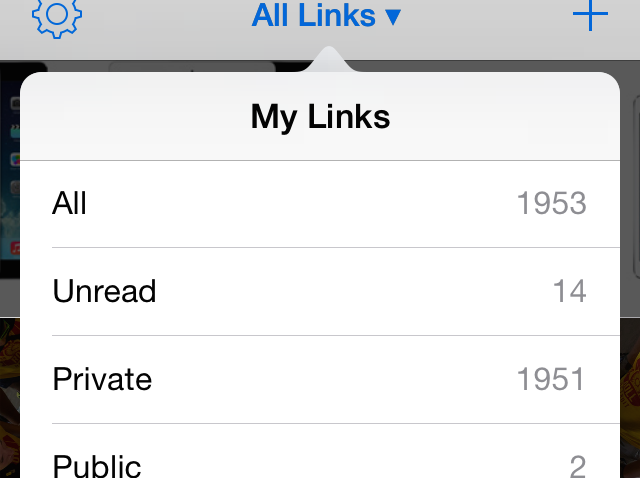
Thankfully it’s easy to force the presentation to be an actual popover. UIPopoverController
is pretty much a thing of the past. With iOS 8 you just present a view controller with a popover presentation style which is a new Adaptive Segue available in Xcode 6.
How to do it
Firstly, your view controller that’s presenting the popover should implement the UIPopoverPresentationControllerDelegate
protocol.
Next, you’ll need to set the popoverPresentationController
’s delegate.
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
// Assuming you've hooked this all up in a Storyboard with a popover presentation style
if ([segue.identifier isEqualToString:@"showPopover"]) {
UINavigationController *destNav = segue.destinationViewController;
PopoverContentsViewController *vc = destNav.viewControllers.firstObject;
// This is the important part
UIPopoverPresentationController *popPC = destNav.popoverPresentationController;
popPC.delegate = self;
}
}
And finally, implement this one little delegate method.
- (UIModalPresentationStyle)adaptivePresentationStyleForPresentationController:(UIPresentationController *)controller {
return UIModalPresentationNone;
}